We will now list down the steps involved for the development of Android based application with backend as MySql, PHP and Xampp Server. Environment selected and Start-up process. We will take a case as catalogue page of shopping cart and mention all the steps involved like Create Activity page, ListAdapter class, AsyncTask class file to get data from server and Layout files.
Creating an Android app requires the SDK (Software Development Kit), an IDE (Integrated Development Environment) like Android Studio or Eclipse, the Java Software Development Kit (JDK) and a virtual device to test on.
Following steps are followed for the development of Application.
- Start Xamp Server
- Start MySql.
- Start Apache Server.
- Use Host Name as follows
- Localhost : to test from Mobile.
- 10.0.2.2 : To test from Module.
- Following activities are performed at Server End.
We will be writing PHP scripts to extract and insert data and the same will be used in android application. The PHP files are stored in directory ./xampp/htdocs/PHP
Images are stored to PHP Server and are loaded. Each Image is named with itemcode and linked to itemcode in database.The Images are stored in directory ./xampp/htdocs/Images.
4. Steps are defined below for Android development Activity.
1. We create an Activity
We Write SQL query . We define a link to PHP script Call the background.class and pass SQL query and PHP link. Get the data from Background AsyncTask in Json data in String Format. Convert the data from Json Data to List<items>.(In List format) Set adapter to ListView as listview.setAdapter(listadapter);2. We create Background AsyncTask Class.
This contains code to execute the PHP Link and get the data in Json format. This contains code to convert json data to List<items>.3. Create Layout file.
Create MainActivity and associate with setContentView(R.layout.activity_list); Create another Layout file for adapter class as new ListAdapter(getBaseContext(), R.layout.list_item, listdata);4. Create Adapter Class that contain Viewholder.5. AndroidManifet.xml is updated as<activity
android:name=".ListActivity"
android:label="@string/title_activity_list" >
</activity>
Environment
- Xampp Server.
- Mysql 5.6
- PHP
- Eclipse – Kepler with android Installed.
Start XAMPP Server
We start the two modules which are required for development.
- Start MySql.
- Start Apache Server.
We can use the option config and Netstat in case of port conflict or server is not starting.
Netstat will show as which ports are used by which application or in idle state. With config option we can change the config script.
Certain ports are blocked by your network admin or ISP.
Perhaps port 80 is already being used by some other application (like IIS) and you don’t want to or are not allowed to shut it down or change it.
Apache Server is not starting. We may change the Apache Server port. This is one way of checking to see if you have a port conflict – change the port and if Apache Server starts working, then you know some other application is using port 80. You should try several ports, just in case you are lucky and manage to pick ports used by other applications. Some ports to try are 2375, 4173, 5107, 9260, 20010
To change the port we Locate the line that says Listen 80. Change to the port to say, 8080. Save the script and start the server again.
Following screen show the XAMPP Control Panel to start MySql and Apache Server.
Write PHP Script to retrieve and insert data into MySql. We will create two scripts – One to establish a connection to database and other to retrieve and insert data to serve.
PHP code lines explain as follows.
First we create connection script to connect to MySql database. We pass the parameter user name, password, localhost and database name to MySql server. This will connect to MySql server.
Second, we set up an SQL query that selects the columns from the table. The next line of code runs the query and puts the resulting data into a variable called $result.
Then, the function num_rows() checks if there are more than zero rows returned.
If there are more than zero rows returned, the function fetch_assoc() puts all the results into an associative array that we can loop through. The while() loop loops through the result set and outputs the data in Json format. We can check the result set on browser.
1. PHP script to establish a connection to database.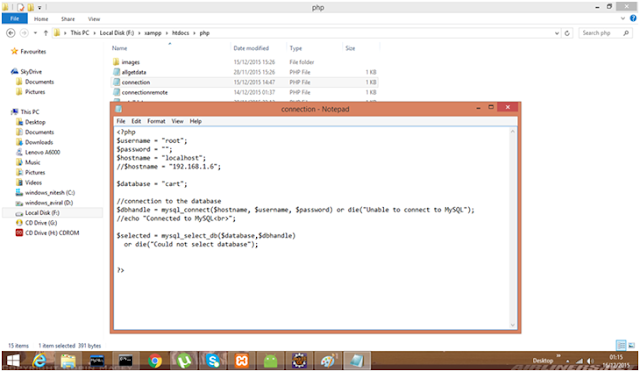
2. PHP Script to retrieve(or insert) data from/to server.
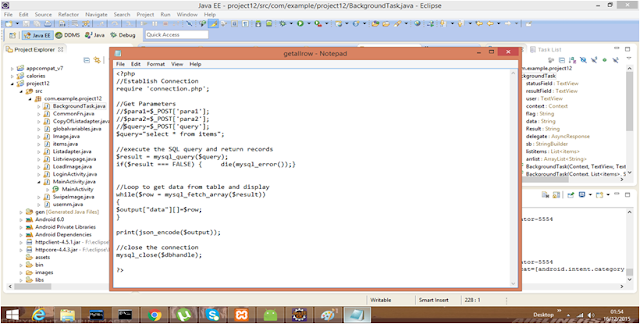
3. Check to see the result in browser by running the PHP Script.
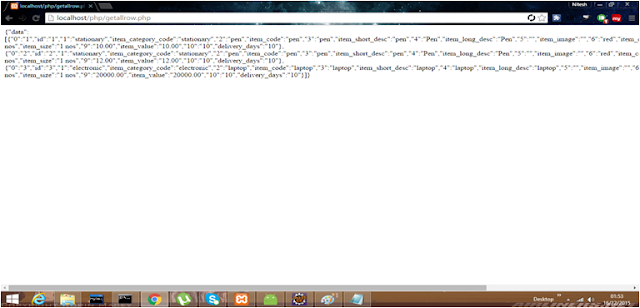
Write AsyncTask class which enables proper and easy use of the UI thread. This class perform background operations and publish results. We will create a class as BackgroundTask.
AsyncTask enables proper and easy use of the UI thread. This class allows to perform background operations and publish results on the UI thread without having to manipulate threads and/or handlers. AsyncTask, The most common methods you will need to implement are onPreExecute(), doInBackground(Params…), onProgressUpdate() andonPostExecute(Result).
The most common methods you will need to implement are these:
1. onPreExecute() – called on the UI thread before the thread starts running. This method is usually used to setup the task, for example by displaying a progress bar.
2. doInBackground(Params…) – this is the method that runs on the background thread. In this method you should put all the code you want the application to perform in background. Referring to our Simple RSS Aplication, you would put here the code that downloads the XML feed and does the parsing. The doInBackground() is called immediately after onPreExecute(). When it finishes, it sends the result to the onPostExecute().
3. onProgressUpdate() – called when you invoke publishProgress() in the doInBackground().
4. onPostExecute(Result) – called on the UI thread after the background thread finishes. It takes as parameter the result received from doInBackground().
Now let us start explaining the Android Code development.
File Name: MainActivity.java
public class ListActivity extends Activity {
ListAdapter listadapter;
List<Items> listdata;
GridView listview;
int num=1;
@Overrideprotected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_list);
listview = (GridView) findViewById(R.id.listviewdetail);
listdata=getdata();
listadapter = new ListAdapter(getBaseContext(), R.layout.list_item, listdata);
listview.setAdapter(listadapter);
}
public List<Items> getdata()
{
List<Items> arrstr = null;
try
{
BackgroundTaskOffers bto = new BackgroundTaskOffers();
// String host="10.0.2.2"; //host for mobile emulator other wise server host name
// String host="192.168.1.7:8080";
String host=((GlobalVariable) getApplicationContext()).getHost();
String query="Select * from items";
String link ="http://"+host+"/php/gettabledata.php";
String[] fields = {"id","item_code","item_short_desc","item_color","item_size","item_value"};
String str = bto.execute(query,link).get();
arrstr = bto.getItems(str,fields);
}catch(Exception e)
{e.printStackTrace();Log.v("system error","**");}
return arrstr;
}
}
BackgroundTask Class File Name : BackgroundTaskOffers.java
public class BackgroundTaskOffers extends AsyncTask<String, Void, String> {
String Result;
StringBuilder sb = new StringBuilder();
@Overrideprotected String doInBackground(String... arg0) {
String query = (String) arg0[0];
String link = (String) arg0[1];
Result = link(query, link);
return Result;
}
public String link(String query, String link) {
try {
String data = URLEncoder.encode("query", "UTF-8") + "="
+ URLEncoder.encode(query, "UTF-8");
URL url = new URL(link);
URLConnection conn = url.openConnection();
conn.setDoOutput(true);
OutputStreamWriter wr = new OutputStreamWriter(
conn.getOutputStream());
wr.write(data);
wr.flush();
String line = null;
BufferedReader reader = new BufferedReader(new InputStreamReader(
conn.getInputStream()));
while ((line = reader.readLine()) != null) {
sb.append(line);
}
} catch (Exception e) {
e.printStackTrace();
}
return sb.toString();
}
// get the data in array format for adapter. jsondata is passed with database fields.
// This method is for Items details adapter and show data in ListView.
public List<Items> getItems(String jsondata, String[] data) {
List<Items> allitems = new LinkedList<Items>();
try {
JSONObject jobject = new JSONObject(jsondata);
JSONArray jmain = jobject.optJSONArray("data");
for (int i = 0; i < jmain.length(); i++) {
JSONObject jchild = jmain.getJSONObject(i);
Items items = new Items();
items.setId(Integer.parseInt(jchild.optString(data[0])));
items.setItem_code(jchild.optString(data[1]));
items.setItem_short_desc(jchild.optString(data[2]));
items.setItem_color(jchild.optString(data[3]));
items.setItem_size(jchild.optString(data[4]));
items.setItem_value(Double.parseDouble(jchild
.optString(data[5])));
allitems.add(items);
}
} catch (JSONException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return allitems;
}
ListAdapter Class File Name: ListAdapter.java
package com.example.cart;
import java.util.List;import java.util.concurrent.ExecutionException;
import com.example.cart.R.drawable;
import android.app.Activity;
import android.app.Dialog;
import android.content.Context;
import android.graphics.Color;
import android.graphics.drawable.Drawable;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class ListAdapter extends ArrayAdapter<Items> {
Context contextm;
GlobalVariable gv = new GlobalVariable();
public ListAdapter(Context context, int resource, List<Items> items) {
super(context, resource, items);
this.contextm = context;
}
public class ViewHolder {
TextView txtid;
TextView txtitemcode;
TextView txtitemshortdesc;
TextView txtitemcolor;
TextView txtitemsize;
TextView txtitemvalue;
ImageView imageview;
Button cartbut1;
}
public View getView(int position, View contentview, ViewGroup parent) {
final ViewHolder holder;
LayoutInflater inflater = (LayoutInflater) contextm
.getSystemService(Activity.LAYOUT_INFLATER_SERVICE);
final Items rowitem = (Items) getItem(position);
if (contentview == null) {
//contentview = inflater.inflate(R.layout.list_item, null);
contentview = inflater.inflate(R.layout.list_item, null);
holder = new ViewHolder();
holder.txtid = (TextView) contentview.findViewById(R.id.txtid);
holder.txtitemcode = (TextView) contentview
.findViewById(R.id.txtitemcode);
holder.txtitemshortdesc = (TextView) contentview
.findViewById(R.id.txtitemshortdesc);
holder.txtitemcolor = (TextView) contentview
.findViewById(R.id.txtitemcolor);
holder.txtitemsize = (TextView) contentview
.findViewById(R.id.txtitemsize);
holder.txtitemvalue = (TextView) contentview
.findViewById(R.id.txtitemvalue);
holder.imageview = (ImageView) contentview
.findViewById(R.id.imageview);
holder.cartbut1 = (Button) contentview
.findViewById(R.id.cartbtn);
contentview.setTag(holder);
holder.cartbut1.setOnClickListener( new OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
fn_insertaddtocart(holder.txtitemcode.getText().toString(),"abc");
}
});
} else
holder = (ViewHolder) contentview.getTag();
holder.txtid.setText(String.valueOf(rowitem.getId()));
holder.txtitemcode.setText(rowitem.getItem_code());
holder.txtitemshortdesc.setText(rowitem.getItem_short_desc());
holder.txtitemcolor.setText(rowitem.getItem_color());
holder.txtitemsize.setText(rowitem.getItem_size());
holder.txtitemvalue.setText(String.valueOf(rowitem.getItem_value()));
String host = gv.getHost();
String url = gv.getImageUrl();
String imageurl = "http://" + host + url + rowitem.getItem_code()
+ ".jpg";
new BackgroundTaskImage(contextm, holder.imageview).execute(imageurl);
if (position % 2 == 1)
contentview.setBackgroundColor(Color.GRAY);
return contentview;
}
public void fnbtn() {
Log.v("error", "button");
}
private void fn_insertaddtocart(String itemcode,String shopper) {
String user = ((GlobalVariable) contextm.getApplicationContext()).getUserName();
BackgroundTaskLogin bg_login = new BackgroundTaskLogin();
try {
String query =
"insert into schedule(usercode,item_code,shopper,schedule_order,schedule_type,schedule_date)"
+ " select '"
+ user +"','"
+ itemcode + "','"
+shopper +"',"
+"schedule_mas.schedule_order,"
+"schedule_mas.schedule_type,"
+"if(schedule_mas.schedule_order='1',curdate(),null)"
+" from schedule_mas";
String link = "http://"
+ ((GlobalVariable) contextm.getApplicationContext()).getHost()
+ "/php/insertdata.php";
Log.v("error", query);
String str = bg_login.execute(query, link).get();
String msg=bg_login.insertusr(str);
Log.v("error1", msg);
CToast ct = new CToast();
ct.showToast(contextm.getApplicationContext(), "Added to Cart, View Cart");
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (ExecutionException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Layout File : Activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.cart.ListActivity" >
<LinearLayout
android:id="@+id/layout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<LinearLayout
android:id="@+id/MenuBar2"
android:layout_width="match_parent"
android:layout_height="30sp"
android:background="#000000"
android:orientation="horizontal" >
<ImageView
android:id="@+id/imagesize"
android:layout_width="30sp"
android:layout_height="30sp"
android:onClick="fnimagesize"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/imagesize" />
<ImageView
android:id="@+id/gridsize"
android:layout_width="30sp"
android:layout_height="30sp"
android:onClick="fngrid"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/grid" />
<ImageView
android:id="@+id/up"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_marginLeft="200sp"
android:onClick="up"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/up" />
<ImageView
android:id="@+id/down"
android:layout_width="30sp"
android:layout_height="30sp"
android:onClick="down"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/down" />
</LinearLayout>
<HorizontalScrollView
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:id="@+id/listview2"
android:layout_width="1000sp"
android:layout_height="410sp"
android:layout_marginTop="2sp"
android:orientation="horizontal"
android:scrollbars="horizontal">
<GridView
android:id="@+id/listviewdetail"
android:layout_width="400dp"
android:numColumns="3"
android:layout_height="420sp"
android:background="@drawable/greenstyle"
/>
</LinearLayout>
</HorizontalScrollView>
</LinearLayout>
<Button
android:id="@+id/prevbut"
android:layout_width="100sp"
android:layout_height="35sp"
android:layout_alignParentBottom="true"
android:layout_marginLeft="100sp"
android:background="@drawable/redstyle"
style="@style/whitebold"
android:onClick="prevpage"
android:text="Previous" />
</RelativeLayout>
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.cart.ListActivity" >
<LinearLayout
android:id="@+id/layout1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<LinearLayout
android:id="@+id/MenuBar2"
android:layout_width="match_parent"
android:layout_height="30sp"
android:background="#000000"
android:orientation="horizontal" >
<ImageView
android:id="@+id/imagesize"
android:layout_width="30sp"
android:layout_height="30sp"
android:onClick="fnimagesize"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/imagesize" />
<ImageView
android:id="@+id/gridsize"
android:layout_width="30sp"
android:layout_height="30sp"
android:onClick="fngrid"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/grid" />
<ImageView
android:id="@+id/up"
android:layout_width="30sp"
android:layout_height="30sp"
android:layout_marginLeft="200sp"
android:onClick="up"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/up" />
<ImageView
android:id="@+id/down"
android:layout_width="30sp"
android:layout_height="30sp"
android:onClick="down"
android:scaleType="fitXY"
android:background="@drawable/greenstyle"
android:padding="4sp"
android:src="@drawable/down" />
</LinearLayout>
<HorizontalScrollView
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:id="@+id/listview2"
android:layout_width="1000sp"
android:layout_height="410sp"
android:layout_marginTop="2sp"
android:orientation="horizontal"
android:scrollbars="horizontal">
<GridView
android:id="@+id/listviewdetail"
android:layout_width="400dp"
android:numColumns="3"
android:layout_height="420sp"
android:background="@drawable/greenstyle"
/>
</LinearLayout>
</HorizontalScrollView>
</LinearLayout>
<Button
android:id="@+id/prevbut"
android:layout_width="100sp"
android:layout_height="35sp"
android:layout_alignParentBottom="true"
android:layout_marginLeft="100sp"
android:background="@drawable/redstyle"
style="@style/whitebold"
android:onClick="prevpage"
android:text="Previous" />
</RelativeLayout>
Layout of above XML
![[activity_main.xml%255B5%255D.png]](https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEgHYZtRJgGRAxtJYxIPZSlgL08SM75bHOwYUwV3S5SrLcKwYFWzoXtxJV1OSeKAhRciZcOSsxlWRl9_DJBuz6I_L83yTZqdSM9cxe9MNd6HJT8oeem5L42xlVB1hyiC3wPUJ0HEu0sWtbq3/s1600/activity_main.xml%25255B5%25255D.png)
Layout File : list_items.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content" >
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
>
<TextView
android:id="@+id/txtid"
style="@style/white"
android:layout_width="10sp"
android:textColor="#000"
android:layout_height="match_parent" />>
<ImageViewandroid:id="@+id/imageview"
android:layout_width="95sp"
android:layout_height="95sp"
android:padding="2sp"
android:scaleType="fitXY" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="120sp"
android:layout_marginLeft="20sp"
android:orientation="vertical" >
<TextView
android:id="@+id/txtitemcode"
style="@style/white"
android:layout_width="50sp"
android:textColor="#000"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/txtitemshortdesc"
style="@style/white"
android:layout_width="150sp"
android:textColor="#000"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/txtitemcolor"
style="@style/white"
android:layout_width="50sp"
android:textColor="#000"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/txtitemsize"
style="@style/white"
android:layout_width="50sp"
android:textColor="#000"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/txtitemvalue"
style="@style/white"
android:layout_width="80sp"
android:textColor="#000"
android:layout_height="wrap_content" />
<Button
android:id="@+id/cartbtn"
style="@style/white"
android:layout_width="70sp"
android:layout_height="30sp"
android:background="@drawable/redstyle"
android:text="Add to Cart" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.cart"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="14"
android:targetSdkVersion="21" />
<application
android:name=".GlobalVariable"
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Image are stored to PHP Server and are loaded. Each Image is named with itemcode and linked to itemcode in database.
We create two directories in ./XAMPP/htdocs as php and images (within PHP). ‘php’ directory will have all php files and images will have all images.
Data will be listed in ListView as shown in following screen.
One can see the complete development of pages on Tutorials Page.
![]() | ![]() | ![]() |